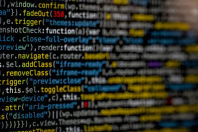
In this tutorial, we will guide you through developing a basic Java application that showcases fundamental programming concepts. This step-by-step guide is tailored for beginners and those looking to refresh their Java skills, with a focus on object-oriented programming (OOP), data structures, and user input/output (I/O).
Learning Objectives
By the end of this tutorial, you will:
- Understand the principles of object-oriented programming in Java.
- Be able to utilize arrays and lists for data storage and manipulation.
- Implement basic user input/output functionality.
- Be capable of writing clear comments and documentation for Java code.
- Know how to create, compile, and execute Java programs.
Tools and Setup
Before we begin, ensure you have the following tools installed:
- Java Development Kit (JDK): Required for compiling and running Java programs.
- Integrated Development Environment (IDE): Recommended IDEs include IntelliJ IDEA, Eclipse, or NetBeans.
- JUnit: For unit testing.
- Mockito: For creating mock objects.
- Selenium: For integration testing.
Key Features and Code Examples
1. Object-Oriented Programming Principles
Example Class Definition
Here’s a simple example demonstrating the definition of a class in Java:
Person.java
“`java
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public void displayInfo() {
System.out.println(“Name: ” + name + “, Age: ” + age);
}
}
“`
Inheritance and Polymorphism
Employee.java
“`java
public class Employee extends Person {
private String role;
public Employee(String name, int age, String role) {
super(name, age);
this.role = role;
}
@Override
public void displayInfo() {
super.displayInfo();
System.out.println(“Role: ” + role);
}
}
“`
2. Data Structures Use (Arrays and Lists)
Arrays Example
ArrayExample.java
“`java
public class ArrayExample {
public static void main(String[] args) {
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println(number);
}
}
}
“`
Lists Example
ListExample.java
“`java
import java.util.ArrayList;
import java.util.List;
public class ListExample {
public static void main(String[] args) {
List<String> fruits = new ArrayList<>();
fruits.add(“Apple”);
fruits.add(“Banana”);
fruits.add(“Cherry”);
for (String fruit : fruits) {
System.out.println(fruit);
}
}
}
“`
3. User Input/Output Functionality
UserIOExample.java
“`java
import java.util.Scanner;
public class UserIOExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print(“Enter your name: “);
String name = scanner.nextLine();
System.out.println(“Hello, ” + name + “!”);
}
}
“`
4. Comments and Documentation Clarity
Commenting Example
DocumentedPerson.java
“`java
/**
- Represents a person with a name and age.
*/
public class DocumentedPerson {
private String name;
private int age;
/**
-
- Constructs a new DocumentedPerson with the given name and age.
*
- @param name The name of the person.
- @param age The age of the person.
*/
public DocumentedPerson(String name, int age) {
this.name = name;
this.age = age;
}
/**
- Displays the person’s name and age.
*/
public void displayInfo() {
System.out.println(“Name: ” + name + “, Age: ” + age);
}
}
“`
Testing the Application
Test Scenarios
- Object-Oriented Programming Principles:
- Test object creation and behavior.
- Verify inheritance and polymorphism.
- Data Structures Use (Arrays and Lists):
- Test insertion, deletion, and retrieval operations.
- User Input/Output Functionality:
- Test response to user inputs.
- Comments and Documentation Clarity:
- Validate accuracy and clarity of comments.
- Overall Application Flow and Integration Testing.
Automated Testing Approach
- JUnit for unit testing individual components.
- Mockito for mocking complex dependencies.
- Selenium for integration testing user input/output.
- Continuous Integration (CI) to automate regular testing.
- Test-Driven Development (TDD) to ensure comprehensive test coverage.
Expected Test Coverage
- Unit Testing
- Integration Testing
- End-to-End Testing
- Edge Case Testing
- Load and Performance Testing
- Security Testing
- Usability Testing
- Compatibility Testing
Educational Content
- Object-Oriented Programming: Understand classes, objects, inheritance, and polymorphism.
- Data Structures: Learn about arrays and lists, their usage, and management.
- User Input/Output: Master console-based I/O operations.
- Comments and Documentation: Write meaningful comments and documentation.
Conclusion
Congratulations on creating and testing your first Java application! This exercise has provided you with a foundation in Java programming and testing methodologies. Keep exploring, experimenting, and building your skills as you continue your programming journey. Happy coding!
- Written by: Torvalds
Leave a Reply